Did you know that 42% of attacks on public-facing systems are based on SQL injection? This threat also extends to internal systems but to a lesser degree (12%).
You might think your website is secure, but what about your database? Imagine a hacker gaining unauthorized access to your database, altering data, or even deleting entire tables. Sounds like a nightmare, right? Well, it’s more common than you think.
SQL Injection attacks can have devastating consequences, from stealing sensitive information to gaining administrative rights on a database server. These attacks are often easily preventable. In this article, we’ll walk you through the latest methods to prevent SQL Injection attacks, ensuring you’re not the next headline for a data breach.
So, if you’re still relying on outdated security measures or, worse, have no security measures in place, it’s time to read on. Let’s make sure that your database is a fortress, not an open door.
What is an SQL Injection Attack?
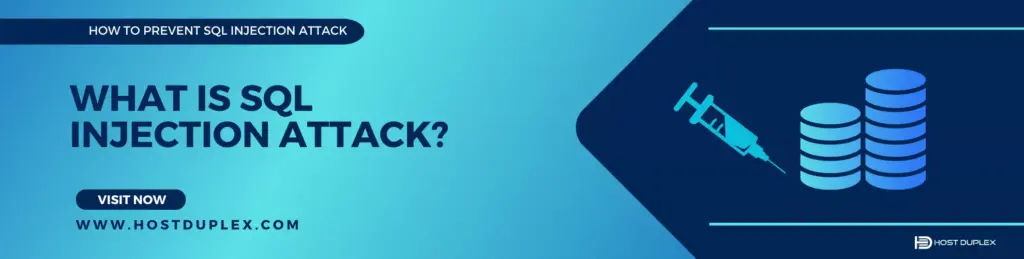
SQL injection is a code injection technique that exploits a vulnerability in a website’s software by manipulating SQL queries. In simpler terms, it’s like tricking the database into revealing, modifying, or deleting data it shouldn’t. This is achieved by inserting malicious SQL statements into input fields for execution.
How Does an SQL Injection Attack Work?
The Role of User Input
SQL injection attacks often start at the application’s user input fields—search bars, login forms, or even URL parameters. These are the points where the application is most vulnerable because it directly interacts with the user.
Malicious SQL Queries
Once the attacker identifies a vulnerable input field, they insert malicious SQL queries. These queries can range from simple commands that drop tables to more complex queries that grant administrative access.
Database Manipulation
The ultimate goal of an SQL injection attack is to manipulate the database. This could mean extracting sensitive customer data, altering pricing information, or even deleting entire databases.
To understand how SQL Injection works, let’s take a look at the following sequence diagram:
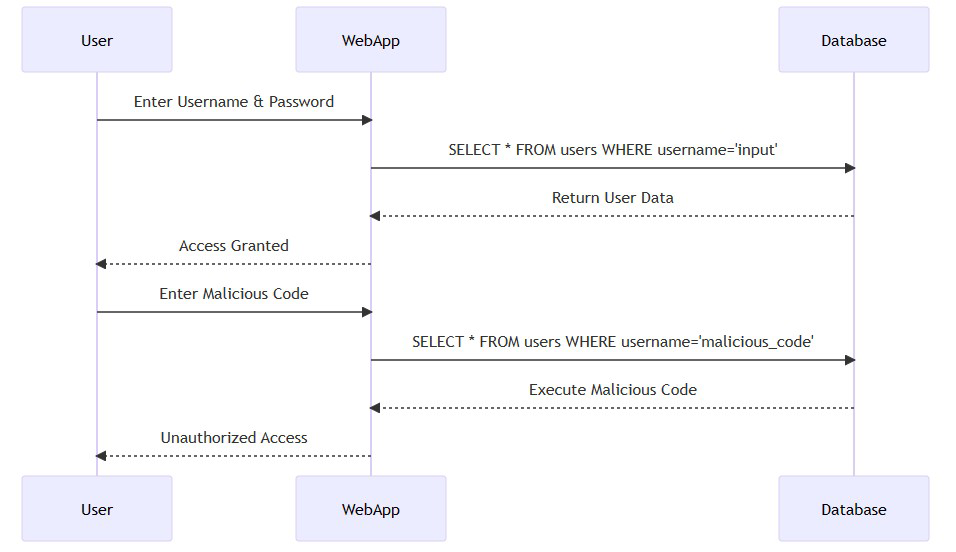
- User: The end-user who interacts with the web application.
- WebApp: The web application that takes user input for authentication.
- Database: The backend SQL server database where user data is stored.
- The User enters their username and password into the WebApp.
- The WebApp constructs an SQL query using the input and sends it to the SQL Database.
- The Database returns the user data to the WebApp, which then grants access to the User.
- The User enters malicious SQL code into the WebApp.
- The WebApp constructs another SQL query using the malicious input and sends it to the SQL server Database.
- The Database executes the malicious SQL code, leading to unauthorized access.
Example of SQL injection attack
Let’s consider a simple login form that takes a username and password. The backend SQL query that verifies the user might look something like this:
SELECT * FROM users WHERE username = '[username]' AND password = '[password]'
Attack
An attacker could input the following in the username field:
‘ OR '1'='1
The SQL query would then become:
SELECT * FROM users WHERE username = '' OR '1'='1' AND password = '[password]'
Result
Because ‘1’=’1′ is always true, this modified SQL statement would return all rows from the users table, effectively bypassing the authentication and potentially giving the attacker unauthorized access to the application.
Types of SQL Injection Attacks
Understanding the types of SQL injection attacks can help you better defend against them. Here are the most common ones:
- Classic SQLi: This is the most straightforward type, where the attacker directly inserts malicious SQL code into a query.
- Blind or Inference SQLi: In this type, the attacker asks the database a true or false question and determines the answer based on the application’s response.
- Time-based Blind SQLi: Similar to Blind SQLi, the attacker determines if the hypothesis is true based on how long it takes the database to respond.
- Error-based SQLi: In Error-based SQL injections, the attacker deliberately triggers database errors to gather information.
How to Identify SQL Injection Vulnerabilities?
The first step in combating SQL injection attacks is knowing how to detect the SQL injection vulnerabilities within your system. So, let’s explore how you can identify these weak points.
Common Signs and Symptoms
- Unexpected Database Errors: If your application starts throwing database errors that you can’t attribute to code changes or server issues, it’s time to investigate SQL injection vulnerabilities.
- Unusual User Activity: Keep an eye on user activity logs. Multiple failed login attempts or strange query patterns could be signs of an attempted SQL injection attack.
- Data Leakage: If you notice that sensitive data is being exposed in your application logs or error messages, this could be a red flag.
Diagnostic Tools and Software
Don’t rely solely on manual checks; use diagnostic tools to automate the process.
- OWASP ZAP: This is an open-source security testing tool used for finding vulnerabilities in web applications. It has specific features for detecting SQL injection vulnerabilities.
- SQLmap: This is another open-source tool that automates the process of detecting and exploiting SQL injection flaws.
SQL Injection Risk Assessment
Automated Scanning Tools
- OWASP ZAP: Not only does it find vulnerabilities, but it also provides you with a risk assessment, helping you prioritize which issues to tackle first.
- SQLmap: Along with detection, SQLmap can also provide a risk assessment based on the vulnerabilities it finds.
Manual Testing Methods
- Code Review: Sometimes, the best way to find vulnerabilities is by manually reviewing the code. Look for instances where user input is directly used in SQL queries without validation.
- Penetration Testing: This involves simulating an SQL injection attack on your application to see how it responds. It’s a hands-on approach that can provide valuable insights into your application’s security posture.
How to Prevent SQL Injection Attacks?
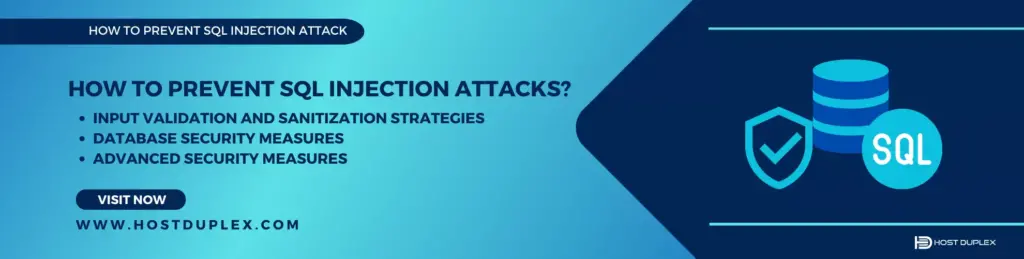
Prevention is better than cure, especially when the cure could cost you millions and tarnish your reputation. Here’s a comprehensive guide to SQL injection prevention techniques.
Input Validation and Sanitization Strategies
Implement Input Filters
Whitelisting: The Proactive Approach
Whitelisting is not just about allowing certain types of data; it’s about creating a stringent set of rules that define what is acceptable. For example, if a field is meant for phone numbers, then only numerical values and perhaps a few special characters like ‘-‘ or ‘+’ should be allowed.
Implement whitelisting by using regular expressions to match patterns of acceptable data. This ensures that any data not fitting the pattern is automatically rejected, making it difficult for attackers to inject malicious SQL code.
Blacklisting: The Reactive Measure
While whitelisting is proactive, blacklisting is more reactive. It involves creating a list of known bad inputs or characters and blocking them. For instance, blocking inputs containing SQL keywords like ‘SELECT,’ ‘DROP,’ etc. However, blacklisting is not foolproof. Skilled attackers can often bypass blacklists using encoding techniques or by exploiting other vulnerabilities. Therefore, use blacklisting only as a supplementary measure, not as your primary defence.
Use Parameterized Queries and Prepared Statements
Parameterized queries are your best friend when it comes to SQL injection prevention. They separate SQL code from the data, ensuring that an attacker can’t manipulate the queries.
Code Examples in Various Languages
In PHP, you can use PDO (PHP Data Objects) to prepare a statement like this:
$stmt = $pdo->prepare('SELECT * FROM users WHERE email = ?');
$stmt->execute([$email]);
In Python, using the psycopg2 library, you can prepare a statement as follows:
cursor.execute("SELECT * FROM users WHERE email = %s", (email,))
In Java, the PreparedStatement class allows you to create parameterized queries:
PreparedStatement ps = connection.prepareStatement("SELECT * FROM users WHERE email = ?");
ps.setString(1, email);
ResultSet rs = ps.executeQuery();
Database Security Measures
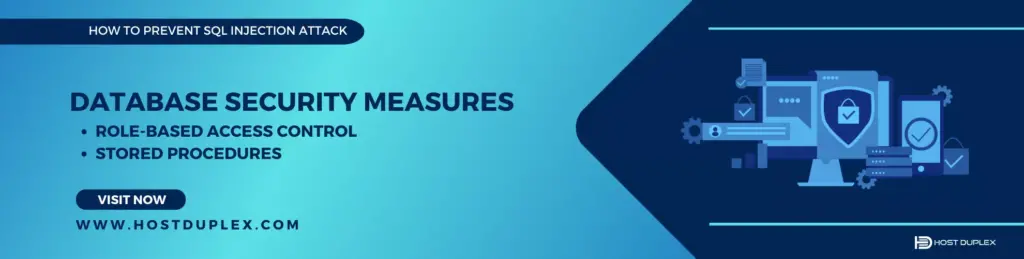
Database security is not just about keeping unauthorized users out; it’s also about limiting what authorized users can do.
Role-Based Access Control
Limit Database Permissions
Not every user or even every administrator needs full access to the database. Role-based access control (RBAC) is a security model where different database users have permissions appropriate to their role within the organization. For example, a ‘read-only’ role might only have permission to execute SELECT queries, while an ‘admin’ role might have more extensive permissions. Implement RBAC by creating roles in your database management system and assigning them appropriate permissions.
Implement Firewalls
A database firewall acts as a gatekeeper, monitoring all incoming and outgoing SQL queries. Configure your firewall to block queries that look suspicious, or that violate your organization’s data access policies. This adds an extra layer of security that can stop an attack even if other defenses fail.
Stored Procedures
Stored procedures are pre-compiled SQL statements stored in the database. They abstract the SQL code, making it harder for attackers to inject malicious SQL.
How to Create and Use Stored Procedures?
To create a stored procedure, you’ll need to use your database management system’s specific syntax. Once created, you can call the stored procedure from your application, passing in parameters as needed. This enhances security and improves performance, as the SQL code is pre-compiled.
Advanced Security Measures
While basic security measures form the foundation of your database security, advanced security measures are the fortified walls that provide an extra layer of protection. These are not just optional add-ons but essential components that can significantly enhance your database’s resilience against SQL injection attacks and other cybersecurity threats.
Use Web Application Firewalls (WAF)
Web Application Firewalls (WAFs) act as a shield between your web application and the internet. They filter and monitor HTTP traffic between a web application and the internet, providing an additional layer of security that can help detect and prevent SQL injection attacks.
Recommended WAF Solutions
Solutions like Cloudflare and Sucuri are highly recommended for their robust features and ease of implementation.
Encrypt Sensitive Information
Always encrypt sensitive data, both at rest and in transit. Encrypting sensitive information ensures that the data remains unintelligible even if an attacker gains access to your database. Use strong encryption algorithms like AES-256 to safeguard your data. Remember, encryption is not just for data at rest; also consider encrypting data in transit using protocols like SSL/TLS.
Conduct Regular Security Audits
Regular security audits are like your periodic health check-ups; they help diagnose issues before they become critical. Use tools like Nessus or OpenVAS for scheduled vulnerability scans. These scans should be comprehensive, covering everything from your database to the web application and network infrastructure.
Be Prepared and Have Incident Response Plans
When a security incident occurs, time is of the essence. A well-structured incident response plan can guide your team through the necessary steps to effectively contain and mitigate the incident.
How Hosting Providers Can Help Prevent SQL Injection Attacks
The role of your hosting provider in website security is often underestimated. While the primary responsibility for preventing SQL injection attacks lies with web developers, a robust hosting environment can offer additional protection. Here’s how:
1. Web Application Firewalls (WAFs)
Many hosting providers offer Web Application Firewalls that are specifically designed to monitor HTTP traffic between a web application and the Internet. These firewalls can identify and filter out malicious SQL queries, providing an initial line of defense against SQL injection attacks.
2. Real-time Monitoring and Alerts
Some hosting services come with real-time monitoring and alert systems that notify you of any suspicious activities on your website. This can include multiple failed login attempts, unexpected data changes, or patterns that match known SQL injection techniques.
3. Regular Software Updates
Hosting providers often take care of server maintenance, including software updates. By keeping the server software and database management systems up-to-date, they help patch any known vulnerabilities that could be exploited through SQL injection.
4. SSL Certificates
While SSL certificates don’t directly protect against SQL injection, they do encrypt the data being sent to and from your website. This adds an extra layer of security, making it more difficult for attackers to intercept or alter data during transmission.
5. Backup Services
In the unfortunate event that your website does get compromised, having a recent backup can be a lifesaver. Many hosting providers like Host Duplex offer automated backup services that allow you to restore your website to a previous, uncompromised state.
6. Isolated Environments
Some premium hosting services offer isolated environments for each hosted application. This isolation prevents a potential SQL injection attack from spreading from one application to another, thereby limiting the damage.
7. Technical Support
Last but not least, a reliable hosting provider will offer 24/7 technical support. If you suspect any malicious activity, immediate technical assistance can be invaluable in preventing or mitigating an attack.

Ongoing Maintenance and Monitoring
The lack of proper maintenance can lead to outdated security measures, while inadequate monitoring can result in unnoticed security breaches.
Keep Your Database and Software Updated
Patch Management
New vulnerabilities are discovered regularly, and patches are released to fix them. Implement a robust patch management system that automatically updates your database software and any related applications. This ensures that you are protected against known vulnerabilities.
Update Schedules
Don’t just update your systems randomly. Have a well-defined schedule for updates and stick to it. Inform your team and stakeholders about upcoming updates to minimize disruptions. Scheduled updates not only keep your system secure but also allow you to allocate resources efficiently.
Set Real-Time Monitoring and Alerts
Intrusion Detection Systems (IDS)
Intrusion Detection Systems (IDS) are like the security cameras of your database. They monitor real-time traffic and alert you to any suspicious activities. Solutions like Snort or Suricata can provide comprehensive monitoring capabilities. These tools can detect SQL injection attempts, unauthorized access, and other malicious activities, allowing you to take immediate action.
Log Analysis
Logs are the footprints left behind by users and systems. Analyzing these logs can provide valuable insights into your database’s security posture. Tools like Splunk or Logstash can help you sift through logs to identify unusual patterns or signs of a security breach.
Bottom Line
SQL injection attacks are a persistent threat, but they’re not invincible. Understanding the anatomy of these attacks and implementing a multi-layered defense strategy can protect your database. But remember, cybersecurity is not a one-time setup; it’s an ongoing process. As the web source highlights, continuous security monitoring is crucial for real-time visibility into your security posture.
Don’t wait for a security breach to happen to take action. Be proactive. Implement the prevention techniques discussed in this article and invest in continuous security monitoring tools.
What is SQL Injection and How to Prevent SQL Injection Attacks (2023)